// DemoTexture.cpp : Implements the CDemoTexture class.
//
// By Geelix School of Serious Games and Edutainment.
//
#include "DemoTexture.h"
// Vertex struct
struct Vertex //버텍스 구조체의 구조를 변경해야함,
{
XMFLOAT3 pos;
XMFLOAT2 tex0; //텍스쳐 내부의 좌표를 참조 x와 y만 있으면 됨.
//정점의 색상을 텍스쳐의 데이터로 바꿔야하기때문에 참조
};
//////////////////////////////////////////////////////////////////////
// Constructors
CDemoTexture::CDemoTexture()
{
m_pVS = NULL;
m_pPS = NULL;
m_pInputLayout = NULL;
m_pVertexBuffer = NULL;
m_pColorMap = NULL;
m_pColorMapSampler = NULL;
}
CDemoTexture::~CDemoTexture()
{
}
//////////////////////////////////////////////////////////////////////
// Overrides
bool CDemoTexture::LoadContent()
{
// Compile vertex shader
ID3DBlob* pVSBuffer = NULL;
bool res = CompileShader(L"ShaderTexture.fx", "VS_Main", "vs_4_0", &pVSBuffer);
if (res == false) {
::MessageBox(m_hWnd, L"Unable to load vertex shader", L"ERROR", MB_OK);
return false;
}
// Create vertex shader
HRESULT hr;
hr = m_pD3DDevice->CreateVertexShader(
pVSBuffer->GetBufferPointer(),
pVSBuffer->GetBufferSize(),
0, &m_pVS);
if (FAILED(hr)) {
if (pVSBuffer)
pVSBuffer->Release();
return false;
}
// Define input layout
D3D11_INPUT_ELEMENT_DESC shaderInputLayout[] =
{
{ "POSITION", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, 0, D3D11_INPUT_PER_VERTEX_DATA, 0 },
//input 레이아웃에 텍스쳐 좌표를 추가해야함.....
{ "TEXCOORD", 0, DXGI_FORMAT_R32G32_FLOAT, 0, 12, D3D11_INPUT_PER_VERTEX_DATA, 0 }
};
UINT numLayoutElements = ARRAYSIZE(shaderInputLayout);
// Create input layout
hr = m_pD3DDevice->CreateInputLayout(
shaderInputLayout, numLayoutElements,
pVSBuffer->GetBufferPointer(),
pVSBuffer->GetBufferSize(),
&m_pInputLayout);
if (FAILED(hr)) {
return false;
}
// Release VS buffer
pVSBuffer->Release();
pVSBuffer = NULL;
// Compile pixel shader
ID3DBlob* pPSBuffer = NULL;
res = CompileShader(L"ShaderTexture.fx", "PS_Main", "ps_4_0", &pPSBuffer);
if (res == false) {
::MessageBox(m_hWnd, L"Unable to load pixel shader", L"ERROR", MB_OK);
return false;
}
// Create pixel shader
hr = m_pD3DDevice->CreatePixelShader(
pPSBuffer->GetBufferPointer(),
pPSBuffer->GetBufferSize(),
0, &m_pPS);
if (FAILED(hr)) {
return false;
}
// Cleanup PS buffer
pPSBuffer->Release();
pPSBuffer = NULL;
// Define triangle
Vertex vertices[] =
{
//사각형은 두개의 삼각형을 이용함.
//정점의 좌표와 텍스쳐 이미지의 좌표를 저장한다. 텍스쳐이미지는 2D이기때문에 2차원 좌표계 이용
{ XMFLOAT3( 0.4f, 0.5f, 1.0f ), XMFLOAT2( 1.0f, 1.0f ) }, //우측 하단 모서리
{ XMFLOAT3( 0.4f, -0.5f, 1.0f ), XMFLOAT2( 1.0f, 0.0f ) }, //우측 상단
{ XMFLOAT3( -0.4f, -0.5f, 1.0f ), XMFLOAT2( 0.0f, 0.0f ) }, //좌측 상단
{ XMFLOAT3( -0.4f, -0.5f, 1.0f ), XMFLOAT2( 0.0f, 0.0f ) },
{ XMFLOAT3( -0.4f, 0.5f, 1.0f ), XMFLOAT2( 0.0f, 1.0f ) },
{ XMFLOAT3( 0.4f, 0.5f, 1.0f ), XMFLOAT2( 1.0f, 1.0f ) },
};
// Vertex description
D3D11_BUFFER_DESC vertexDesc;
::ZeroMemory(&vertexDesc, sizeof(vertexDesc));
vertexDesc.Usage = D3D11_USAGE_DEFAULT;
vertexDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexDesc.ByteWidth = sizeof(Vertex) * 6;
// Resource data
D3D11_SUBRESOURCE_DATA resourceData;
ZeroMemory(&resourceData, sizeof(resourceData));
resourceData.pSysMem = vertices;
// Create vertex buffer
hr = m_pD3DDevice->CreateBuffer(&vertexDesc, &resourceData, &m_pVertexBuffer);
if (FAILED(hr)) {
return false;
}
// Load texture
// DDS파일을 불러온다.
hr = ::D3DX11CreateShaderResourceViewFromFile(
m_pD3DDevice, L"borg.dds", 0, 0, &m_pColorMap, 0);
if (FAILED(hr)) {
::MessageBox(m_hWnd, L"Unable to load texture", L"ERROR", MB_OK);
return false;
}
// Texture sampler
// 텍스쳐 샘플러는 질감을 샘플링함..(여러 기능이 있음)
D3D11_SAMPLER_DESC textureDesc;
::ZeroMemory(&textureDesc, sizeof(textureDesc));
textureDesc.AddressU = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.AddressV = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.AddressW = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.ComparisonFunc = D3D11_COMPARISON_NEVER;
textureDesc.Filter = D3D11_FILTER_MIN_MAG_MIP_LINEAR;
textureDesc.MaxLOD = D3D11_FLOAT32_MAX;
hr = m_pD3DDevice->CreateSamplerState(&textureDesc, &m_pColorMapSampler);
if (FAILED(hr)) {
::MessageBox(m_hWnd, L"Unable to create texture sampler state", L"ERROR", MB_OK);
return false;
}
return true;
}
void CDemoTexture::UnloadContent()
{
// Cleanup
if (m_pColorMap)
m_pColorMap->Release();
m_pColorMap = NULL;
if (m_pColorMapSampler)
m_pColorMapSampler->Release();
m_pColorMapSampler = NULL;
if (m_pVS)
m_pVS->Release();
m_pVS = NULL;
if (m_pPS)
m_pPS->Release();
m_pPS = NULL;
if (m_pInputLayout)
m_pInputLayout->Release();
m_pInputLayout = NULL;
if (m_pVertexBuffer)
m_pVertexBuffer->Release();
m_pVertexBuffer = NULL;
}
void CDemoTexture::Update()
{
}
void CDemoTexture::Render()
{
// Check if D3D is ready
if (m_pD3DContext == NULL)
return;
// Clear back buffer
float color[4] = { 0.0f, 0.0f, 0.5f, 1.0f };
m_pD3DContext->ClearRenderTargetView(m_pD3DRenderTargetView, color);
// Stride and offset
UINT stride = sizeof(Vertex);
UINT offset = 0;
// Set vertex buffer
m_pD3DContext->IASetInputLayout(m_pInputLayout);
m_pD3DContext->IASetVertexBuffers(0, 1, &m_pVertexBuffer, &stride, &offset);
m_pD3DContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
// Set shaders
m_pD3DContext->VSSetShader(m_pVS, 0, 0);
m_pD3DContext->PSSetShader(m_pPS, 0, 0);
//ShaderTexture.fx속의 변수들을 설정한다.
//컬러맵, 컬러샘플 선언
//Texture2D colorMap : register(t0);
//SamplerState colorSampler : register(s0);
m_pD3DContext->PSSetShaderResources(0, 1, &m_pColorMap);
m_pD3DContext->PSSetSamplers(0, 1, &m_pColorMapSampler);
// Draw triangles
m_pD3DContext->Draw(6, 0);
// Present back buffer to display
m_pSwapChain->Present(0, 0);
}
// ShaderTexture.fx : Implements the shader.
//
// By Geelix School of Serious Games and Edutainment.
//
//컬러맵, 컬러샘플 선언
Texture2D colorMap : register(t0);
SamplerState colorSampler : register(s0);
struct VS_Input
{
float4 pos : POSITION;
float2 tex0 : TEXCOORD0;
};
struct PS_Input
{
float4 pos : SV_POSITION;
float2 tex0 : TEXCOORD0;
};
PS_Input VS_Main(VS_Input vertex)
{
PS_Input vsOut = (PS_Input)0;
vsOut.pos = vertex.pos;
vsOut.tex0 = vertex.tex0;
return vsOut;
}
//텍스쳐가 실제로 출력되는곳
float4 PS_Main(PS_Input frag) : SV_TARGET
{
return colorMap.Sample(colorSampler, frag.tex0);
}
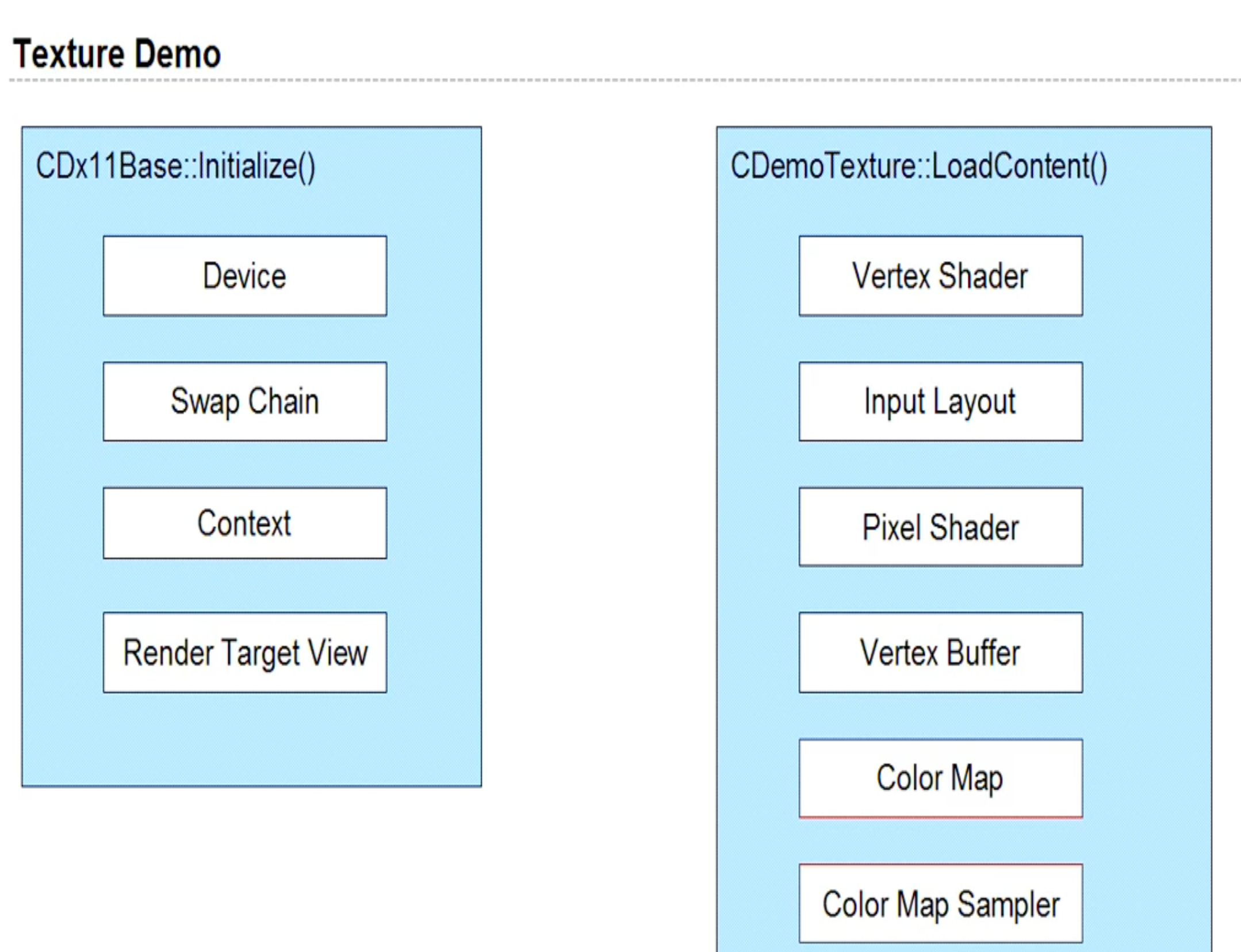
'기타 > directX' 카테고리의 다른 글
[DirectX11] 삼각형 그리기 (0) | 2022.02.26 |
---|---|
[DirectX11] 렌더링 (0) | 2022.02.26 |
[DirectX11] sprite 그리기 (0) | 2022.02.26 |
// DemoTexture.cpp : Implements the CDemoTexture class.
//
// By Geelix School of Serious Games and Edutainment.
//
#include "DemoTexture.h"
// Vertex struct
struct Vertex //버텍스 구조체의 구조를 변경해야함,
{
XMFLOAT3 pos;
XMFLOAT2 tex0; //텍스쳐 내부의 좌표를 참조 x와 y만 있으면 됨.
//정점의 색상을 텍스쳐의 데이터로 바꿔야하기때문에 참조
};
//////////////////////////////////////////////////////////////////////
// Constructors
CDemoTexture::CDemoTexture()
{
m_pVS = NULL;
m_pPS = NULL;
m_pInputLayout = NULL;
m_pVertexBuffer = NULL;
m_pColorMap = NULL;
m_pColorMapSampler = NULL;
}
CDemoTexture::~CDemoTexture()
{
}
//////////////////////////////////////////////////////////////////////
// Overrides
bool CDemoTexture::LoadContent()
{
// Compile vertex shader
ID3DBlob* pVSBuffer = NULL;
bool res = CompileShader(L"ShaderTexture.fx", "VS_Main", "vs_4_0", &pVSBuffer);
if (res == false) {
::MessageBox(m_hWnd, L"Unable to load vertex shader", L"ERROR", MB_OK);
return false;
}
// Create vertex shader
HRESULT hr;
hr = m_pD3DDevice->CreateVertexShader(
pVSBuffer->GetBufferPointer(),
pVSBuffer->GetBufferSize(),
0, &m_pVS);
if (FAILED(hr)) {
if (pVSBuffer)
pVSBuffer->Release();
return false;
}
// Define input layout
D3D11_INPUT_ELEMENT_DESC shaderInputLayout[] =
{
{ "POSITION", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, 0, D3D11_INPUT_PER_VERTEX_DATA, 0 },
//input 레이아웃에 텍스쳐 좌표를 추가해야함.....
{ "TEXCOORD", 0, DXGI_FORMAT_R32G32_FLOAT, 0, 12, D3D11_INPUT_PER_VERTEX_DATA, 0 }
};
UINT numLayoutElements = ARRAYSIZE(shaderInputLayout);
// Create input layout
hr = m_pD3DDevice->CreateInputLayout(
shaderInputLayout, numLayoutElements,
pVSBuffer->GetBufferPointer(),
pVSBuffer->GetBufferSize(),
&m_pInputLayout);
if (FAILED(hr)) {
return false;
}
// Release VS buffer
pVSBuffer->Release();
pVSBuffer = NULL;
// Compile pixel shader
ID3DBlob* pPSBuffer = NULL;
res = CompileShader(L"ShaderTexture.fx", "PS_Main", "ps_4_0", &pPSBuffer);
if (res == false) {
::MessageBox(m_hWnd, L"Unable to load pixel shader", L"ERROR", MB_OK);
return false;
}
// Create pixel shader
hr = m_pD3DDevice->CreatePixelShader(
pPSBuffer->GetBufferPointer(),
pPSBuffer->GetBufferSize(),
0, &m_pPS);
if (FAILED(hr)) {
return false;
}
// Cleanup PS buffer
pPSBuffer->Release();
pPSBuffer = NULL;
// Define triangle
Vertex vertices[] =
{
//사각형은 두개의 삼각형을 이용함.
//정점의 좌표와 텍스쳐 이미지의 좌표를 저장한다. 텍스쳐이미지는 2D이기때문에 2차원 좌표계 이용
{ XMFLOAT3( 0.4f, 0.5f, 1.0f ), XMFLOAT2( 1.0f, 1.0f ) }, //우측 하단 모서리
{ XMFLOAT3( 0.4f, -0.5f, 1.0f ), XMFLOAT2( 1.0f, 0.0f ) }, //우측 상단
{ XMFLOAT3( -0.4f, -0.5f, 1.0f ), XMFLOAT2( 0.0f, 0.0f ) }, //좌측 상단
{ XMFLOAT3( -0.4f, -0.5f, 1.0f ), XMFLOAT2( 0.0f, 0.0f ) },
{ XMFLOAT3( -0.4f, 0.5f, 1.0f ), XMFLOAT2( 0.0f, 1.0f ) },
{ XMFLOAT3( 0.4f, 0.5f, 1.0f ), XMFLOAT2( 1.0f, 1.0f ) },
};
// Vertex description
D3D11_BUFFER_DESC vertexDesc;
::ZeroMemory(&vertexDesc, sizeof(vertexDesc));
vertexDesc.Usage = D3D11_USAGE_DEFAULT;
vertexDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexDesc.ByteWidth = sizeof(Vertex) * 6;
// Resource data
D3D11_SUBRESOURCE_DATA resourceData;
ZeroMemory(&resourceData, sizeof(resourceData));
resourceData.pSysMem = vertices;
// Create vertex buffer
hr = m_pD3DDevice->CreateBuffer(&vertexDesc, &resourceData, &m_pVertexBuffer);
if (FAILED(hr)) {
return false;
}
// Load texture
// DDS파일을 불러온다.
hr = ::D3DX11CreateShaderResourceViewFromFile(
m_pD3DDevice, L"borg.dds", 0, 0, &m_pColorMap, 0);
if (FAILED(hr)) {
::MessageBox(m_hWnd, L"Unable to load texture", L"ERROR", MB_OK);
return false;
}
// Texture sampler
// 텍스쳐 샘플러는 질감을 샘플링함..(여러 기능이 있음)
D3D11_SAMPLER_DESC textureDesc;
::ZeroMemory(&textureDesc, sizeof(textureDesc));
textureDesc.AddressU = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.AddressV = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.AddressW = D3D11_TEXTURE_ADDRESS_WRAP;
textureDesc.ComparisonFunc = D3D11_COMPARISON_NEVER;
textureDesc.Filter = D3D11_FILTER_MIN_MAG_MIP_LINEAR;
textureDesc.MaxLOD = D3D11_FLOAT32_MAX;
hr = m_pD3DDevice->CreateSamplerState(&textureDesc, &m_pColorMapSampler);
if (FAILED(hr)) {
::MessageBox(m_hWnd, L"Unable to create texture sampler state", L"ERROR", MB_OK);
return false;
}
return true;
}
void CDemoTexture::UnloadContent()
{
// Cleanup
if (m_pColorMap)
m_pColorMap->Release();
m_pColorMap = NULL;
if (m_pColorMapSampler)
m_pColorMapSampler->Release();
m_pColorMapSampler = NULL;
if (m_pVS)
m_pVS->Release();
m_pVS = NULL;
if (m_pPS)
m_pPS->Release();
m_pPS = NULL;
if (m_pInputLayout)
m_pInputLayout->Release();
m_pInputLayout = NULL;
if (m_pVertexBuffer)
m_pVertexBuffer->Release();
m_pVertexBuffer = NULL;
}
void CDemoTexture::Update()
{
}
void CDemoTexture::Render()
{
// Check if D3D is ready
if (m_pD3DContext == NULL)
return;
// Clear back buffer
float color[4] = { 0.0f, 0.0f, 0.5f, 1.0f };
m_pD3DContext->ClearRenderTargetView(m_pD3DRenderTargetView, color);
// Stride and offset
UINT stride = sizeof(Vertex);
UINT offset = 0;
// Set vertex buffer
m_pD3DContext->IASetInputLayout(m_pInputLayout);
m_pD3DContext->IASetVertexBuffers(0, 1, &m_pVertexBuffer, &stride, &offset);
m_pD3DContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
// Set shaders
m_pD3DContext->VSSetShader(m_pVS, 0, 0);
m_pD3DContext->PSSetShader(m_pPS, 0, 0);
//ShaderTexture.fx속의 변수들을 설정한다.
//컬러맵, 컬러샘플 선언
//Texture2D colorMap : register(t0);
//SamplerState colorSampler : register(s0);
m_pD3DContext->PSSetShaderResources(0, 1, &m_pColorMap);
m_pD3DContext->PSSetSamplers(0, 1, &m_pColorMapSampler);
// Draw triangles
m_pD3DContext->Draw(6, 0);
// Present back buffer to display
m_pSwapChain->Present(0, 0);
}
// ShaderTexture.fx : Implements the shader.
//
// By Geelix School of Serious Games and Edutainment.
//
//컬러맵, 컬러샘플 선언
Texture2D colorMap : register(t0);
SamplerState colorSampler : register(s0);
struct VS_Input
{
float4 pos : POSITION;
float2 tex0 : TEXCOORD0;
};
struct PS_Input
{
float4 pos : SV_POSITION;
float2 tex0 : TEXCOORD0;
};
PS_Input VS_Main(VS_Input vertex)
{
PS_Input vsOut = (PS_Input)0;
vsOut.pos = vertex.pos;
vsOut.tex0 = vertex.tex0;
return vsOut;
}
//텍스쳐가 실제로 출력되는곳
float4 PS_Main(PS_Input frag) : SV_TARGET
{
return colorMap.Sample(colorSampler, frag.tex0);
}
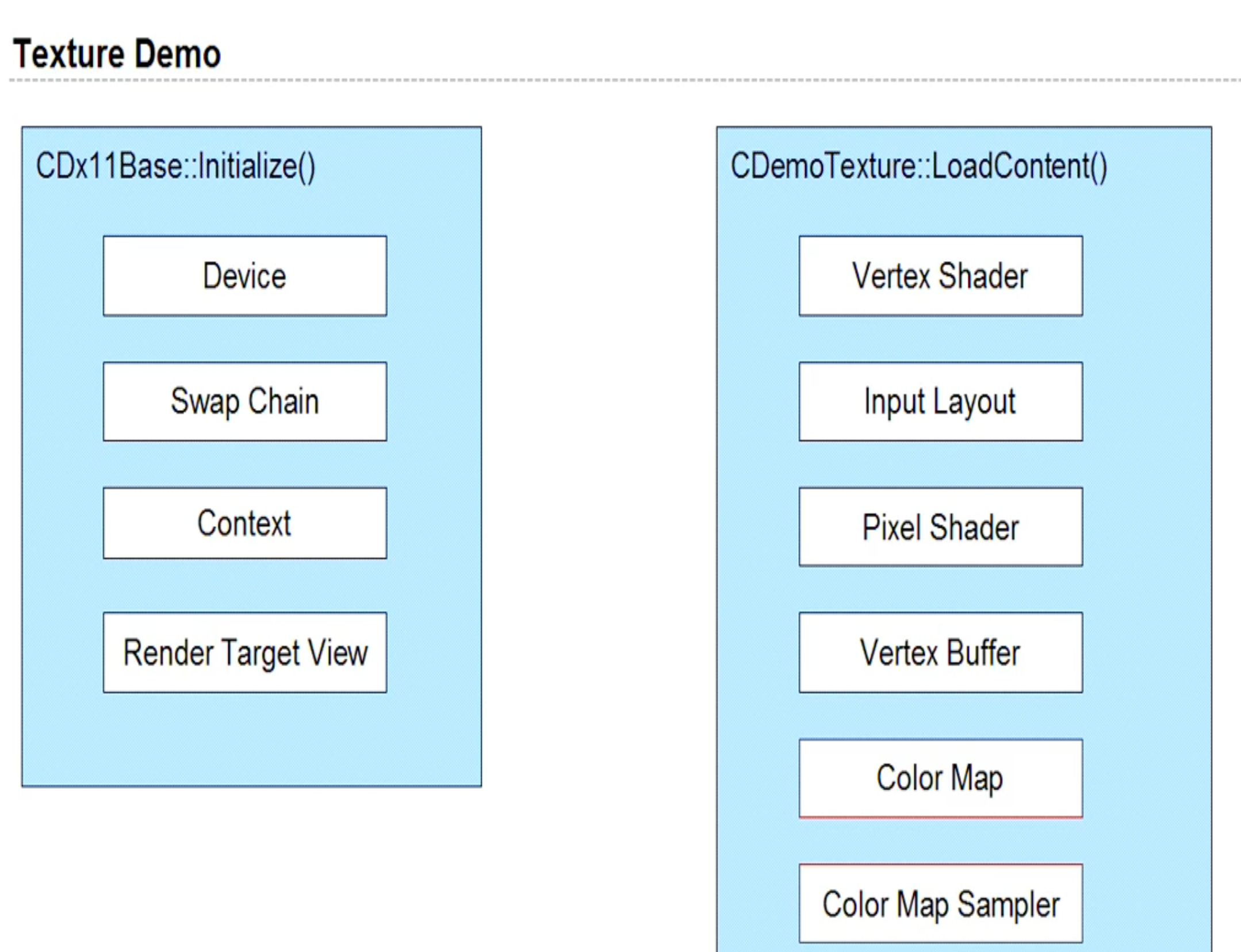
'기타 > directX' 카테고리의 다른 글
[DirectX11] 삼각형 그리기 (0) | 2022.02.26 |
---|---|
[DirectX11] 렌더링 (0) | 2022.02.26 |
[DirectX11] sprite 그리기 (0) | 2022.02.26 |