바인딩 한 변수의 값을 변경하였는데도 화면에서는 바뀌지 않는 경우가 있습니다. 이런 상황에서 강제로 업데이트를 통해 현재의 변수값으로 보여주도록 하는 방법을 정리하였습니다.
· 항상 동기화하지 않는다!
- 바인딩을 한 변수가 변경된다고해서 항상 화면에 업데이트 된 값으로 변경되는것은 아닙니다.
@page "/counter"
@using System.Threading;
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<button class="btn btn-secondary" @onclick="AutoIncrement">Auto Increment</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
private void AutoIncrement()
{
var timer = new Timer(x =>
{
InvokeAsync(() =>
{
IncrementCount();
});
}, null, 1000, 1000);
}
}
- 'Auto Increment' 버튼을 누르면 서브 스레드가 일정시간마다 IncrementCount를 호출하여 currentCount를 1씩 증가시키도록 합니다.
- 하지만 이 상태에서는 AutoIncrement에의해 변경되는 변수값 자체는 바뀌지만 화면에 업데이트되지 않습니다.
· 동기화 안됨
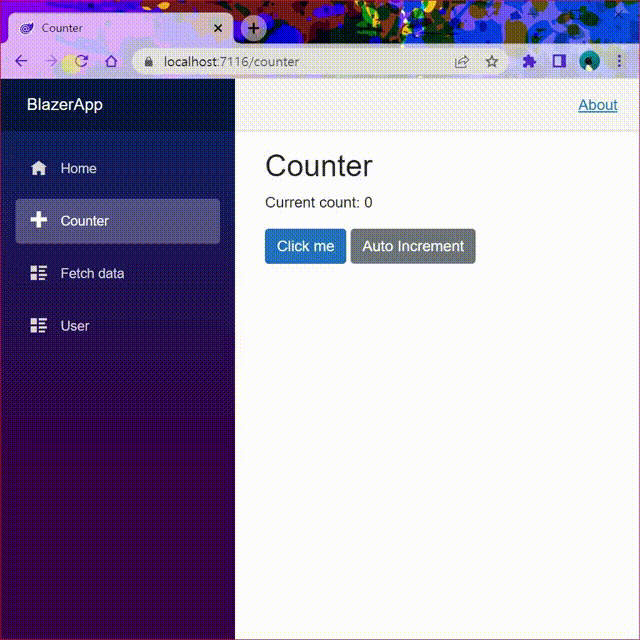
- Click me를 누를때는 Auto Increment에 의해 변경된 값까지 포함하여 변경되는것을 볼 수 있습니다.
- 하지만 Click me를 누르지 않을때는 값이 업데이트되지 않습니다.
· 강제로 업데이트하기
- 원할때 상태가 바뀌었다고 명시적으로 함수를 호출하여 강제로 업데이트를 할 수 있습니다.
- 코드는 다음과 같습니다.
//명시적으로 상태를 변경했다고 알려줌!
StateHasChanged();
더보기
@page "/counter"
@using System.Threading;
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<button class="btn btn-secondary" @onclick="AutoIncrement">Auto Increment</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
private void AutoIncrement()
{
var timer = new Timer(x =>
{
InvokeAsync(() =>
{
IncrementCount();
//명시적으로 상태를 변경했다고 알려줌!
StateHasChanged();
});
}, null, 1000, 1000);
}
}
· 강제 업데이트
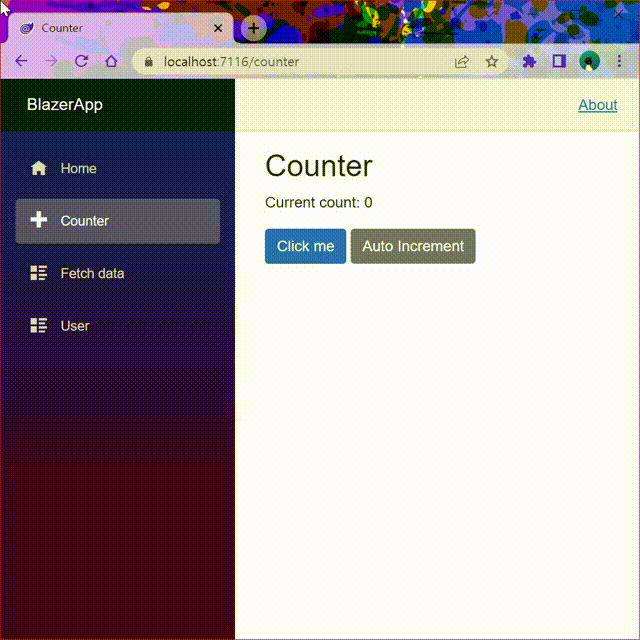
- 이제는 Click me를 누르지 않아도 일정시간마다 1씩 증가하는것을 볼 수 있습니다.
'server > web server' 카테고리의 다른 글
[Web] Cascading 파라미터 (0) | 2023.02.24 |
---|---|
[Web] 컴포넌트 재사용 (0) | 2023.02.24 |
[Web] 속성 바인딩 (0) | 2023.02.23 |
[Web] List 바인딩 (0) | 2023.02.23 |
[Web] 바인딩 (0) | 2023.02.23 |
바인딩 한 변수의 값을 변경하였는데도 화면에서는 바뀌지 않는 경우가 있습니다. 이런 상황에서 강제로 업데이트를 통해 현재의 변수값으로 보여주도록 하는 방법을 정리하였습니다.
· 항상 동기화하지 않는다!
- 바인딩을 한 변수가 변경된다고해서 항상 화면에 업데이트 된 값으로 변경되는것은 아닙니다.
@page "/counter"
@using System.Threading;
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<button class="btn btn-secondary" @onclick="AutoIncrement">Auto Increment</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
private void AutoIncrement()
{
var timer = new Timer(x =>
{
InvokeAsync(() =>
{
IncrementCount();
});
}, null, 1000, 1000);
}
}
- 'Auto Increment' 버튼을 누르면 서브 스레드가 일정시간마다 IncrementCount를 호출하여 currentCount를 1씩 증가시키도록 합니다.
- 하지만 이 상태에서는 AutoIncrement에의해 변경되는 변수값 자체는 바뀌지만 화면에 업데이트되지 않습니다.
· 동기화 안됨
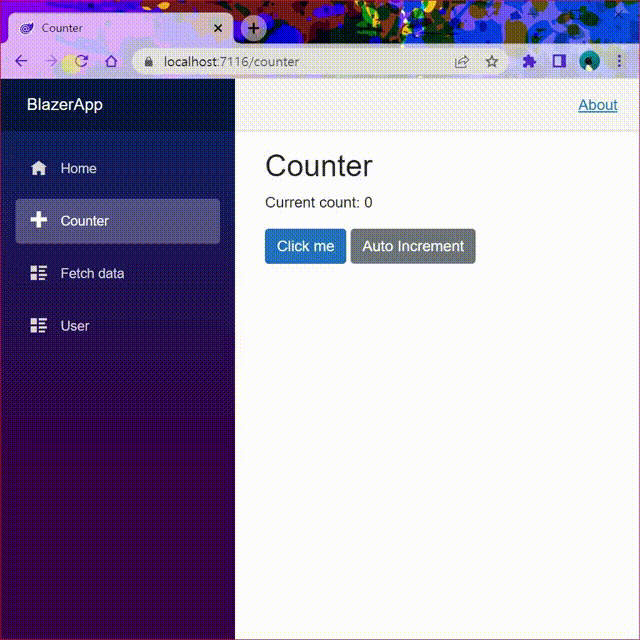
- Click me를 누를때는 Auto Increment에 의해 변경된 값까지 포함하여 변경되는것을 볼 수 있습니다.
- 하지만 Click me를 누르지 않을때는 값이 업데이트되지 않습니다.
· 강제로 업데이트하기
- 원할때 상태가 바뀌었다고 명시적으로 함수를 호출하여 강제로 업데이트를 할 수 있습니다.
- 코드는 다음과 같습니다.
//명시적으로 상태를 변경했다고 알려줌!
StateHasChanged();
업데이트를 포함한 전체 스크립트 보기
@page "/counter"
@using System.Threading;
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<button class="btn btn-secondary" @onclick="AutoIncrement">Auto Increment</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
private void AutoIncrement()
{
var timer = new Timer(x =>
{
InvokeAsync(() =>
{
IncrementCount();
//명시적으로 상태를 변경했다고 알려줌!
StateHasChanged();
});
}, null, 1000, 1000);
}
}
· 강제 업데이트
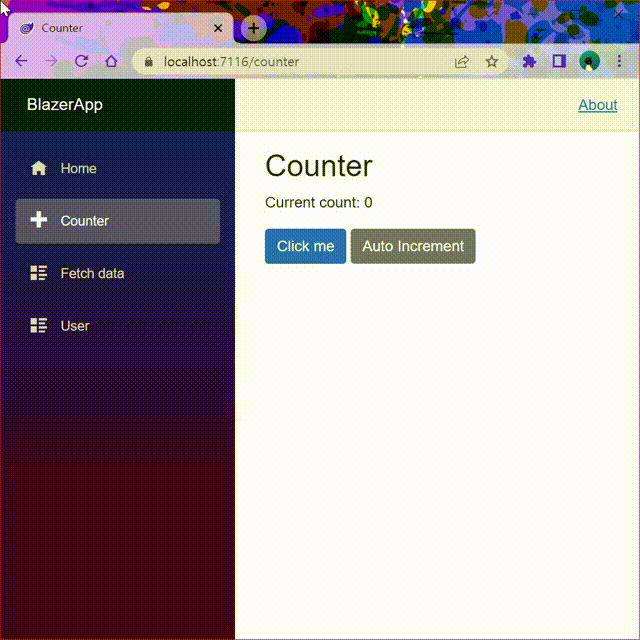
- 이제는 Click me를 누르지 않아도 일정시간마다 1씩 증가하는것을 볼 수 있습니다.
'server > web server' 카테고리의 다른 글
[Web] Cascading 파라미터 (0) | 2023.02.24 |
---|---|
[Web] 컴포넌트 재사용 (0) | 2023.02.24 |
[Web] 속성 바인딩 (0) | 2023.02.23 |
[Web] List 바인딩 (0) | 2023.02.23 |
[Web] 바인딩 (0) | 2023.02.23 |