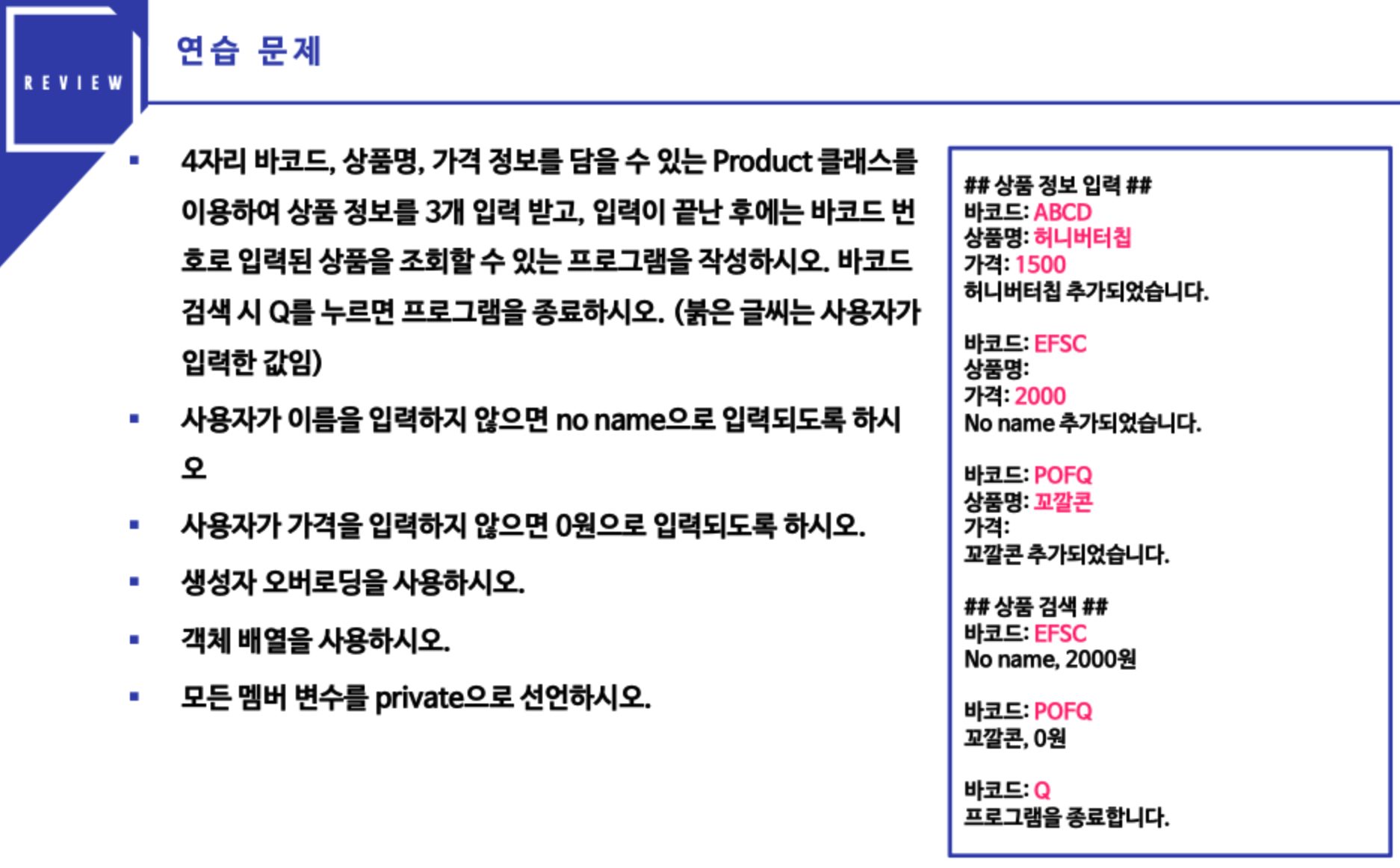
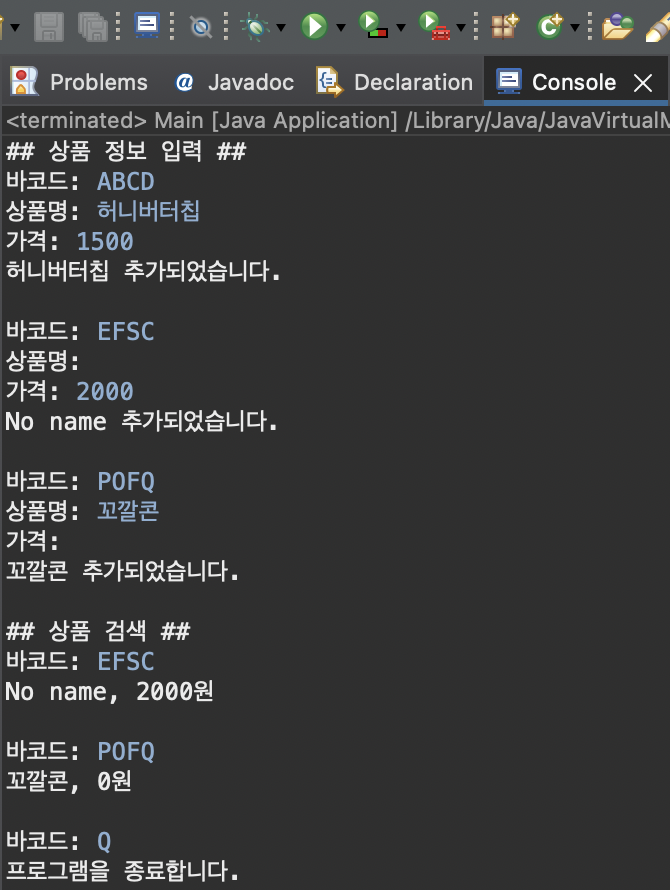
1. Run 할 시, 실행되는 메인 함수
> ProductManager을 생성하며 내부 생성자에서 작업을 처리하도록 한다.
import java.util.Scanner;
public class Main
{
public static Scanner Scanner;
public static void main(String[] args)
{
Scanner = new Scanner(System.in);
new ProductManager();
}
}
|
cs |
2. Product 클래스.
> Product에 담겨질 내용들을 선언하고, 생성자 오버라이드와 게터를 통해 클래스에 접근하도록 하였다.
public class Product
{
private String mBarcode;
private String mProductName;
private int mPrice;
public Product(String barcode, String productName, int price)
{
this.mBarcode = barcode;
this.mProductName = productName;
this.mPrice = price;
}
public Product(String barcode, int price)
{
this(barcode, "No name", price);
}
public Product(String barcode, String productName)
{
this(barcode, productName, 0);
}
public Product(String barcode)
{
this(barcode, "No name", 0);
}
public String GetBarcode() { return mBarcode; }
public String GetProductName() { return mProductName; }
public int GetPrice() { return mPrice; }
}
|
cs |
3. 핵심 기능이 들어있는 ProductManager
> ArrayList로 선언하여 Product가 유동적으로 들어갈 수 있게 하였다.
> 입력란이 공백일 경우가 있으므로 모두 String Type으로 NextLine을 이용해 입력을 받고 입력값이 ""(비어있음)인지 확인하여 적절한 생성자를 호출하도록 구현한것이 핵심이다.
> Java에서는 String 변수의 비교를 String == "..." 으로 하면 참이 나오지 않기에(문자열 자체를 비교하지 않고 메모리를 비교함) 객체 클래스 함수인 .equals("...") 함수를 통해 검색 기능을 구현하였다.
import java.util.ArrayList;
public class ProductManager
{
private ArrayList<Product> mProducts;
public ProductManager()
{
mProducts = new ArrayList<Product>();
this.Start();
}
private void Start()
{
System.out.println("## 상품 정보 입력 ##");
//상품 데이터 입력(3회)
for(int i = 0; i < 3; ++i) { InputProduct(); }
SearchProduct();
System.out.print("프로그램을 종료합니다.");
}
private void InputProduct()
{
String barcode, name, price;
System.out.print("바코드: ");
barcode = Main.Scanner.nextLine();
System.out.print("상품명: ");
name = Main.Scanner.nextLine();
System.out.print("가격: ");
price = Main.Scanner.nextLine();
if(name.equals("") && price.equals(""))
{
mProducts.add(new Product(barcode));
}
else if(name.equals(""))
{
mProducts.add(new Product(barcode, Integer.parseInt(price)));
}
else if(price.equals(""))
{
mProducts.add(new Product(barcode, name));
}
else
{
mProducts.add(new Product(barcode, name, Integer.parseInt(price)));
}
System.out.println(mProducts.get(mProducts.size() - 1).GetProductName() + " 추가되었습니다. \n");
}
private void SearchProduct()
{
String input;
System.out.println("## 상품 검색 ##");
while(true)
{
System.out.print("바코드: ");
input = Main.Scanner.nextLine();
if(input.equals("Q")) { break; }
for(int i = 0; i < mProducts.size(); ++i)
{
if(mProducts.get(i).GetBarcode().equals(input))
{
System.out.println(mProducts.get(i).GetProductName() + ", " + mProducts.get(i).GetPrice() + "원\n");
}
}
}
}
}
|
cs |
'기타 > univ. projects' 카테고리의 다른 글
[유니티] 게임엔진응용실습 Unit2 프로젝트 - 장애물 코스 피하기 게임 (0) | 2022.09.13 |
---|---|
[유니티] 게임엔진응용실습 Unit1 프로젝트 (Unity 기초) (0) | 2022.09.13 |
[자바] 멀티미디어자바프로젝트II ch.03 연습문제 (0) | 2022.09.13 |
[자바] JFrame 블랙잭(BlackJack) 게임 구현 (0) | 2022.07.15 |
[자바] 블랙잭(BlackJack) 게임 구현 (0) | 2022.03.27 |
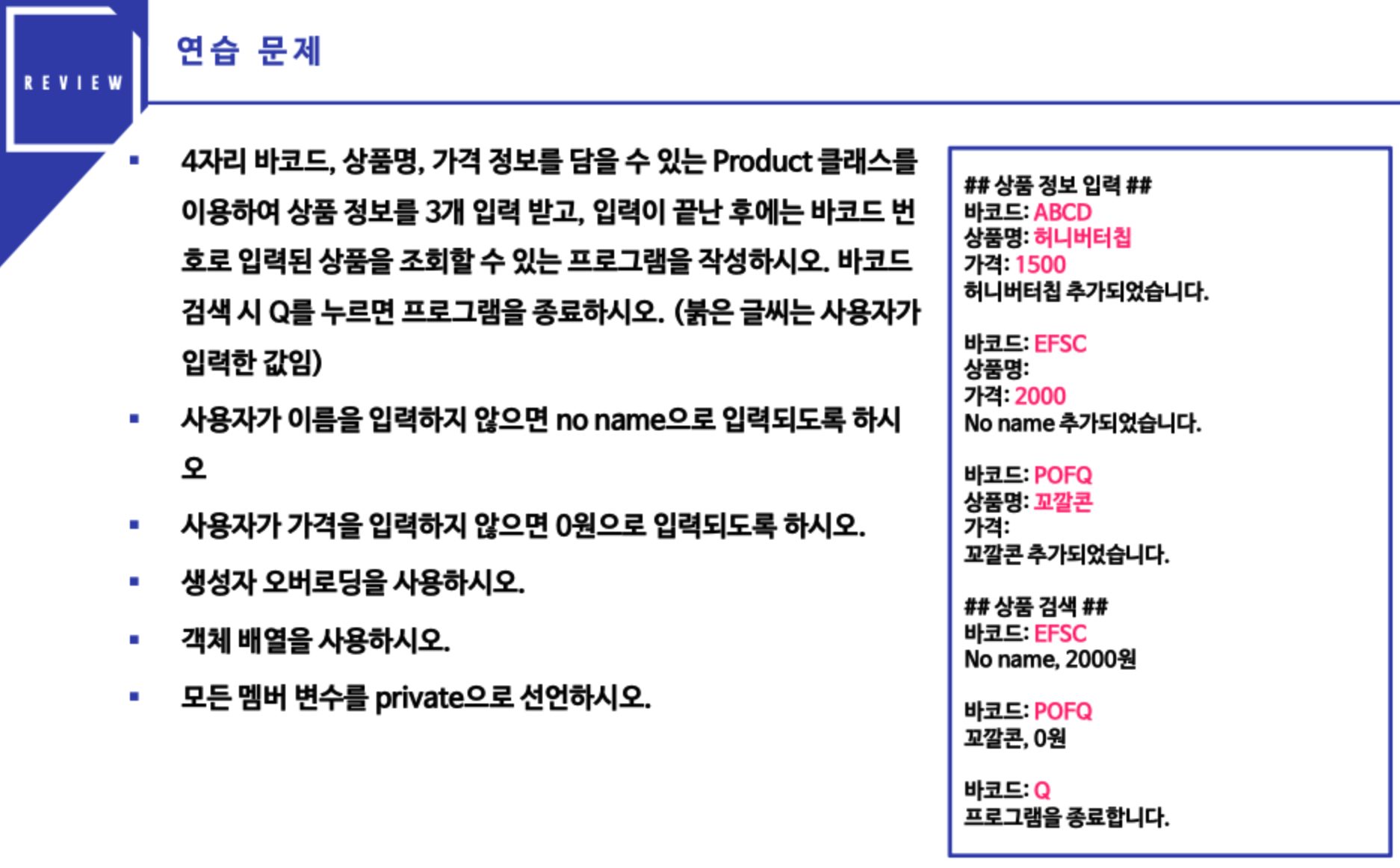
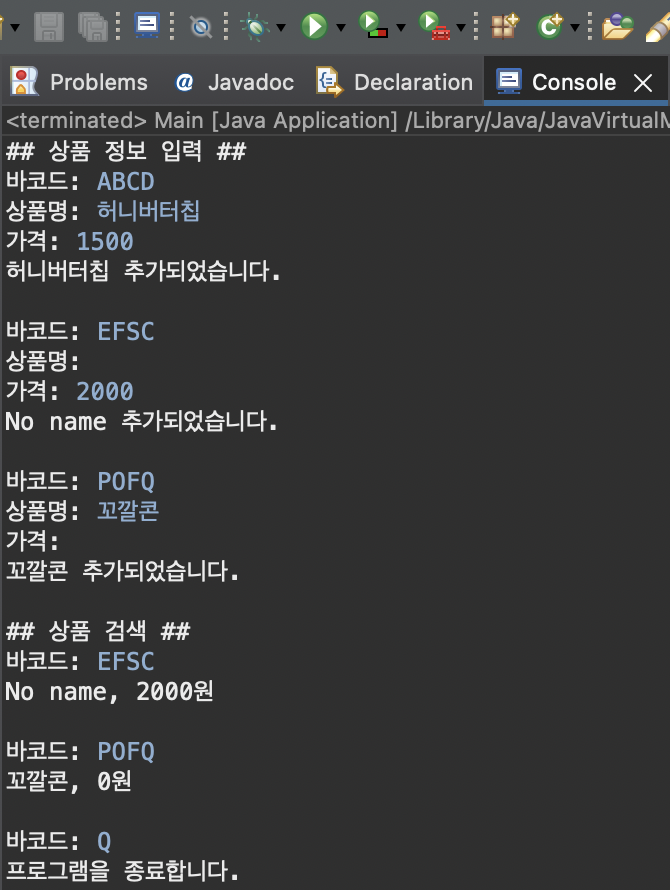
1. Run 할 시, 실행되는 메인 함수
> ProductManager을 생성하며 내부 생성자에서 작업을 처리하도록 한다.
import java.util.Scanner;
public class Main
{
public static Scanner Scanner;
public static void main(String[] args)
{
Scanner = new Scanner(System.in);
new ProductManager();
}
}
|
cs |
2. Product 클래스.
> Product에 담겨질 내용들을 선언하고, 생성자 오버라이드와 게터를 통해 클래스에 접근하도록 하였다.
public class Product
{
private String mBarcode;
private String mProductName;
private int mPrice;
public Product(String barcode, String productName, int price)
{
this.mBarcode = barcode;
this.mProductName = productName;
this.mPrice = price;
}
public Product(String barcode, int price)
{
this(barcode, "No name", price);
}
public Product(String barcode, String productName)
{
this(barcode, productName, 0);
}
public Product(String barcode)
{
this(barcode, "No name", 0);
}
public String GetBarcode() { return mBarcode; }
public String GetProductName() { return mProductName; }
public int GetPrice() { return mPrice; }
}
|
cs |
3. 핵심 기능이 들어있는 ProductManager
> ArrayList로 선언하여 Product가 유동적으로 들어갈 수 있게 하였다.
> 입력란이 공백일 경우가 있으므로 모두 String Type으로 NextLine을 이용해 입력을 받고 입력값이 ""(비어있음)인지 확인하여 적절한 생성자를 호출하도록 구현한것이 핵심이다.
> Java에서는 String 변수의 비교를 String == "..." 으로 하면 참이 나오지 않기에(문자열 자체를 비교하지 않고 메모리를 비교함) 객체 클래스 함수인 .equals("...") 함수를 통해 검색 기능을 구현하였다.
import java.util.ArrayList;
public class ProductManager
{
private ArrayList<Product> mProducts;
public ProductManager()
{
mProducts = new ArrayList<Product>();
this.Start();
}
private void Start()
{
System.out.println("## 상품 정보 입력 ##");
//상품 데이터 입력(3회)
for(int i = 0; i < 3; ++i) { InputProduct(); }
SearchProduct();
System.out.print("프로그램을 종료합니다.");
}
private void InputProduct()
{
String barcode, name, price;
System.out.print("바코드: ");
barcode = Main.Scanner.nextLine();
System.out.print("상품명: ");
name = Main.Scanner.nextLine();
System.out.print("가격: ");
price = Main.Scanner.nextLine();
if(name.equals("") && price.equals(""))
{
mProducts.add(new Product(barcode));
}
else if(name.equals(""))
{
mProducts.add(new Product(barcode, Integer.parseInt(price)));
}
else if(price.equals(""))
{
mProducts.add(new Product(barcode, name));
}
else
{
mProducts.add(new Product(barcode, name, Integer.parseInt(price)));
}
System.out.println(mProducts.get(mProducts.size() - 1).GetProductName() + " 추가되었습니다. \n");
}
private void SearchProduct()
{
String input;
System.out.println("## 상품 검색 ##");
while(true)
{
System.out.print("바코드: ");
input = Main.Scanner.nextLine();
if(input.equals("Q")) { break; }
for(int i = 0; i < mProducts.size(); ++i)
{
if(mProducts.get(i).GetBarcode().equals(input))
{
System.out.println(mProducts.get(i).GetProductName() + ", " + mProducts.get(i).GetPrice() + "원\n");
}
}
}
}
}
|
cs |
'기타 > univ. projects' 카테고리의 다른 글
[유니티] 게임엔진응용실습 Unit2 프로젝트 - 장애물 코스 피하기 게임 (0) | 2022.09.13 |
---|---|
[유니티] 게임엔진응용실습 Unit1 프로젝트 (Unity 기초) (0) | 2022.09.13 |
[자바] 멀티미디어자바프로젝트II ch.03 연습문제 (0) | 2022.09.13 |
[자바] JFrame 블랙잭(BlackJack) 게임 구현 (0) | 2022.07.15 |
[자바] 블랙잭(BlackJack) 게임 구현 (0) | 2022.03.27 |